If you haven’t already would recommend reading an earlier article which spawned me to write this one concerning the SDK differences across CRM Dynamics 2011, 2013, 2015, 2016 and Dynamics 365. If you read that article you would be thinking how the feck I am suppose to write my code to extend CRM with so many different connection options? The issue is that if you get this fundamentally wrong it can lead to abortive code later down the road and a need to potentially re-write your CRM wrapper assemblies.
This article is going to start with the latest version of Dynamics 365 and then work backwards from here so you can see the evolution.
Dynamics 365 and 2016
The End of the CRM SOAP Web Services (nearly… see red crosses!)

You should take note that the 2011 SOAP end point used by the Organisation/Discovery web services are officially deprecated as of CRM 2016; Microsoft are currently in the process of rewriting the SDK assemblies to use Web API instead from Dynamics 365 onwards. This means that the new Web API will be completely replace the Organization/Discovery SOAP based web services. Here is a good article on MSDN about the status of web services.
The current SDK assemblies can still be used for Plugins and Custom Workflow activities which is the recommended approach for Dynamics 365 rather than trying to use Web API within Plugins/CWA. Plus for a time, they can be used for custom applications. Once Microsoft have refactored the SDK assemblies to use Web API you would still continue to use those SDK for Plugin/CWA and then its up to you concerning client applications.
For client applications and indeed the web resources it is recommended that all future application code now uses the Web API (OData 4) which offers all of the capabilities we are use to working with using the OrganizationService SOAP end point. Microsoft have confirmed that in the very near future the SOAP end point will be completely replaced by Web API.
The Web API (OData 4) can be used in both JS and server-side code (e.g. C#) in fact it’s platform independent meaning its easier than ever to integrate with Dynamics 365 from lots of different devices and coding platforms. The other supported way is to connect your custom application to Dynamics 365 by using the SDK Tooling Connector API.
For web resources the OData end point was prevalent in earlier CRM releases but was limited (for example you could do the basic CRUD operations but not execute server messages or FetchXML queries for that you had to create SOAP envelopes and use the organization SOAP end point).
The new Web API can handle the CRUD operations same as before in addition to FetchXML / oData queries as part of the RetrieveMultiple call. Below are the methods now available using the Web API:
- Create
- Update
- Delete
- Retrieve
- Retrieve multiple (using odata query and fetch)
- Execute Web API functions (useful functions which dont change data)
- Execute Web API Actions (parity with the SOAP Execute method to call standard and custom messages for example ExecuteWorkflow)
- Execute Web API Query Functions (Query Options Basically)
Just in case its not yet clear!! The OData (Organisation Data Service) and the SOAP Web Services (Organization and Discovery) are both officially deprecated as of CRM 2016. Although Microsoft will continue to provide extended support for both the Organisation Data Service (OData) the SOAP end points for a period of time as things are progressively rolled out and those customers who need to upgrade CRM have time to refactor there libraries to use Web API.
Dynamics CRM 2015, 2013, 2011

The red blocks are where you would normally see the server name (if on premise) where you are likely to have one or more organisation deployments. For Online its the instance name you have assigned to it via the CRM Admin Centre.
Starting from CRM 2013 onwards the SDK was updated with several assemblies all part of the new XRM Tooling API library:
- Microsoft.Xrm.Tooling.Connector.dll
- Microsoft.Xrm.Tooling.CrmConnectControl.dll
- Microsoft.Xrm.Tooling.CrmConnector.Powershell.dll
- Microsoft.Xrm.Tooling.WebResourceUtility.dll
- Microsoft.Xrm.Tooling.PackageDeployment.Powershell.dll
The intention was that developers would move away from the Microsoft.Xrm.Client.dll assembly (discussed in detail later) and take advantage of the more comprehensive tooling API’s. I can tell you now that for many of my clients who already had an existing CRM Implementation (even upto 2016) most were still using the old Microsoft.Xrm.Client.dll assembly, interestingly enough Microsoft removed it completely from the 2016 and 365 SDK’s. The same thing will no doubt happen concerning SOAP vs. Web API organization and discovery services the best thing developers can do is hit the learning curve sooner.
The tooling API’s basically sit on top of the core assemblies and provide the developer with more options to quickly get applications built that need to integrate with CRM in summary the new tooling assemblies provide:
- Interface for CRM API’s
- Common Login Control (replaces the ConnectionDialog Control)
- Web Resource Utility
- Automated deployment helpers
Microsoft’s Unified Service desk application for example was built using the new set of tooling API’s. That’s great if you are using 2013, 2015 or 2016 but from 2016 onwards (in an ideal world) you should be using the Web API and have re-factored any other code that was dependent on the Organisation Data Service end point (OData) and indeed code that was calling the Organization/Discovery SOAP end points. Existing CRM Online subscribers have a limited time before they will be moved onto the Dynamics 365 platform, so unless that is planned for it could end in disaster especially once the SOAP and OData endpoints are completely removed.
Dynamics 2011 Implementations
There are still many customers out there who are still on Dynamics 2011 and you never know from one CRM Project to the next what version you will be using so its still important to know what is the best approach. Most if not all CRM 2011 implementations will be using the Microsoft.Xrm.Client.dll and more specifically the Microsoft.Xrm.Client.Services namespace within it. Chances are that many will have created there own wrapper class to make the data layer easier to use along with connection caching to improve performance.
Something which is a little confusing at the start and easy to miss is the following:
using Microsoft.Xrm.Sdk.Client;
using Microsoft.Xrm.Client.Services;
These are not the same assemblies.
The first one is part of the main Microsoft.Xrm.Sdk.dll and contains useful classes for proxy connections by including the namespace it means we can define the proxy type as OrganizationService _proxy as opposed to:
Microsoft.Xrm.Sdk.Client.OrganizationServiceProxy _proxy
The other separate assembly Microsoft.Xrm.Client.dll and its .Services namespace is used most of the time, as it contains multiple constructors and classes to make connecting to CRM easier whether that’s for a console application or an Interface based application. For the latter, you may elect to use the ConnectDialog control found in:
Microsoft.Xrm.Client.Windows.Controls.ConnectionDialog namespace.
The services class allows us to instantiate the Organization Service (connection) in a number of different ways:
- Connection string passed via constructor
- Using it’s internal CrmConnection class via constructor
- Pass in a object that has implemented IOrganisation which is normally a proxy object (which itself implements IOrganizationService)
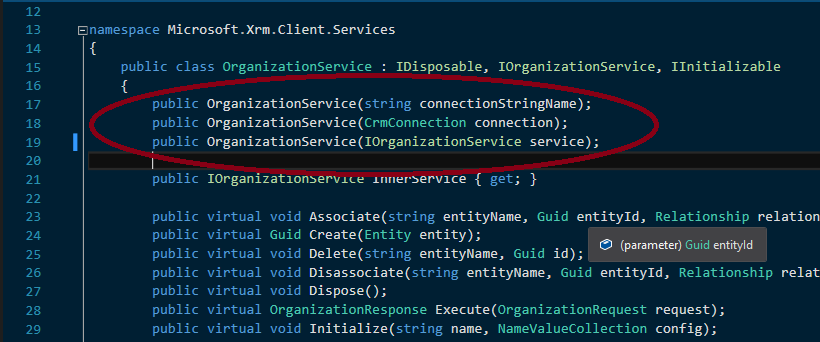
Helper Code
Finally, you could just use the CrmServiceHelpers.cs and DeviceIdManager.cs helper classes found in each of the SDK’s; which pretty much are just referencing the Microsoft.Xrm.Sdk.dll assembly but automatically provide the user with prompts so they can login to multiple CRM server targets and instances/organisations.
Therefore you don’t have to use the Microsoft.Xrm.Client.dll library you could just rely upon the standard assembly. Aimed mostly at 2011-2015 here are some very basic comparisons in code:
Using the Client Services assembly/namespace:
using Microsoft.Xrm.Client.Services;
using Microsoft.Xrm.Sdk;
using Microsoft.Xrm.Sdk.Client;
OrganizationService service;
ClientCredentials MyCredentials = new ClientCredentials();
// you would have to set the properties for MyCredentials first before instantiating your proxy
IOrganizationService _serviceProxy = new OrganizationServiceProxy(new Uri(strURL), null, MyCredentials, null);
service = new OrganizationService(_serviceProxy);
OR
Microsoft.Xrm.Client.CrmConnection connection = CrmConnection.Parse("Url=" + strUrl + "; Domain=" + strDomain + "; Username=" + strUserName + "; Password=" + strPassword + ";");
_service = new OrganizationService(connection);
The approach we see in the SDK examples using the crmhelper classes:
using Microsoft.Xrm.Sdk;
using Microsoft.Xrm.Sdk.Client;
private OrganizationServiceProxy _serviceProxy;
ServerConnection serverConnect = new ServerConnection();
ServerConnection.Configuration config = serverConnect.GetServerConfiguration();
public void Run(ServerConnection.Configuration serverConfig, bool promptforDelete)
{
try
{
using (_serviceProxy =
ServerConnection.GetOrganizationProxy(serverConfig))
}
_serviceProxy.EnableProxyTypes();
_service = (IOrganizationService)_serviceProxy;
}
Depending on the business requirements sometimes we can use the SDK helper classes directly to save a great deal of development time (especially for basic data tools). However most of the time we have classes that are specific to the business itself which requires a combination of the SDK, your custom wrapper class (e.g. myOrgCrmConnection/CRMIntegrator.cs) and the Microsoft.Xrm.Client.Services library.
February 26, 2018 at 12:15 pm
Nice article on Web API and differences: https://community.dynamics.com/enterprise/b/prasannavadlamudiswebblog/archive/2017/09/03/authentication-in-dynamics-365-web-api-and-difference-between-microsoft-dynamics-365-web-api-organization-service-and-organization-data-service
LikeLike
July 22, 2019 at 6:58 am
Thanks for the wonderful article Antony. Please check this article for posting source code. It took me time as well to figure it out https://en.support.wordpress.com/wordpress-editor/blocks/syntax-highlighter-code-block/2/
LikeLiked by 1 person
August 29, 2019 at 9:12 am
Glad you liked it! and thanks for the styling tip I have updated to make this clearer,
LikeLiked by 1 person
June 19, 2020 at 6:45 pm
Hello terrific blog! Does running a blog such as this require a large amount of work? I have virtually no knowledge of programming but I was hoping to start my own blog in the near future. Anyhow, should you have any recommendations or tips for new blog owners please share. I know this is off subject however I just had to ask. Thanks a lot!|
LikeLike